Hey there, you lovely coder! In this post, we’re going to dive deep into the world of Python strings and learn how to compare them like a pro. So, grab a cup of coffee or tea, put on your favorite tunes, and let’s get started!
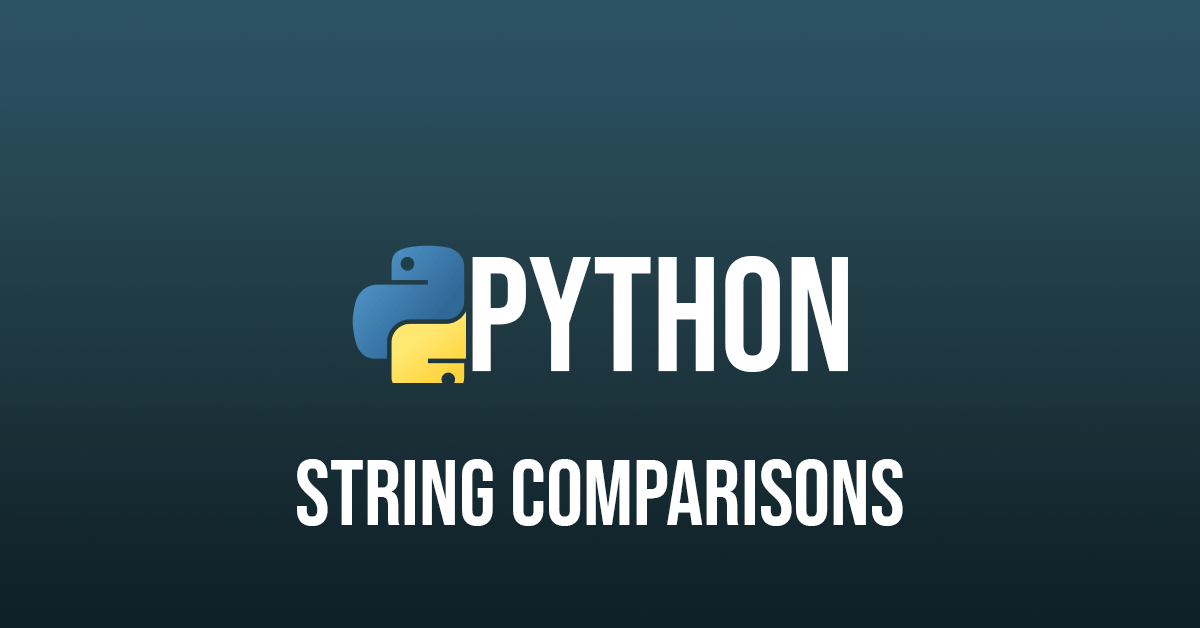
What’s the Deal with Python Strings?
Strings are a basic data type in Python, and they’re a sequence of characters. You can think of them as a row of scrabble tiles or a sentence in a book. But what if you want to compare two strings to see if they’re the same, different, or how they relate to each other? That’s where Python string comparisons come in!
Comparing Strings: The Basics
In Python, we have some operators that allow us to compare strings, like ==
, !=
, <
, >
, <=
, and >=
. When you compare two strings using these operators, Python checks the individual characters’ Unicode values. Let’s see some examples!
The Equality Operators (==
and !=
)
The ==
operator checks if two strings are the same, while the !=
operator checks if they’re different. Here’s some code to give you an idea:
string1 = "apple"
string2 = "banana"
string3 = "apple"
print(string1 == string2) # Output: False
print(string1 == string3) # Output: True
print(string1 != string2) # Output: True
The Comparison Operators (<
, >
, <=
, >=
)
These operators are used to check the order of two strings. They compare strings lexicographically, which means they look at the Unicode values of the individual characters in each string.
string1 = "apple"
string2 = "banana"
string3 = "APPLE"
print(string1 < string2) # Output: True
print(string1 > string3) # Output: True (lowercase letters have higher Unicode values than uppercase letters)
Ignoring Case When Comparing Strings
Sometimes, you don’t care about the case of the strings, and you just want to see if they’re the same, regardless of whether they’re uppercase or lowercase. In that case, you can use the lower()
or upper()
methods to convert both strings to the same case before comparing them.
string1 = "apple"
string2 = "APPLE"
print(string1.lower() == string2.lower()) # Output: True
The in
and not in
Keywords
You can also check if a substring exists in another string using the in
keyword, and the not in
keyword to check if it doesn’t.
string1 = "I love Python!"
print("love" in string1) # Output: True
print("hate" not in string1) # Output: True
Comparing Strings with Built-in Functions
Python has some built-in functions that can help you compare strings, too! Let’s look at the ord()
and chr()
functions, as well as the str.compare()
method (available in Python 3.10 and later).
The ord()
and chr()
Functions
ord()
returns the Unicode value of a single character, while chr()
does the opposite, converting a Unicode value back to its corresponding character.
print(ord("a")) # Output: 97
print(chr(97)) # Output: 'a'
The str.compare()
Method
Starting with Python 3.10, you can use the str.compare()
method to compare two strings. This method returns an integer: a negative value if the first string comes before the second, a positive value if the first string comes after the second, and 0 if both strings are equal.
string1 = "apple"
string2 = "banana"
string3 = "apple"
print(str.compare(string1, string2)) # Output: -1
print(str.compare(string1, string3)) # Output: 0
print(str.compare(string2, string1)) # Output: 1
String Comparisons with Custom Sorting
What if you need to compare strings in a more specific way, like sorting a list of strings based on length, ignoring non-alphabetic characters, or something else entirely? That’s where custom sorting comes in handy!
Sorting Strings by Length
If you want to sort a list of strings based on their length, you can use the sorted()
function with a custom key
argument. Check this out:
words = ["apple", "banana", "cherry", "kiwi", "mango"]
sorted_words = sorted(words, key=len)
print(sorted_words) # Output: ['kiwi', 'apple', 'mango', 'banana', 'cherry']
Ignoring Non-Alphabetic Characters
If you need to compare strings while ignoring non-alphabetic characters, you can use a custom sorting function. In this example, we’ll create a function that removes non-alphabetic characters from a string and use it as a key
argument in the sorted()
function.
import re
def remove_non_alpha(string):
return re.sub(r"[^a-zA-Z]", "", string)
strings = ["apple!", "ba#na@na", "c$he^rr&y"]
sorted_strings = sorted(strings, key=remove_non_alpha)
print(sorted_strings) # Output: ['apple!', 'ba#na@na', 'c$he^rr&y']
Conclusion
That’s a wrap, folks! We’ve explored the world of Python string comparisons, from basic operators like ==
and !=
to custom sorting using the sorted()
function. We’ve seen how to ignore case when comparing strings, use the in
and not in
keywords, and work with Python’s built-in functions like ord()
and chr()
. We even touched on the str.compare()
method available in Python 3.10 and later.
I hope you enjoyed this chill and informal guide to Python string comparisons. Now go out there and start comparing strings like a boss! And remember, keep it cool and keep it fun, because that’s what coding is all about. 🐍✌️